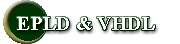
EPLD Circuit -
Altera EPM7064SLC44-10
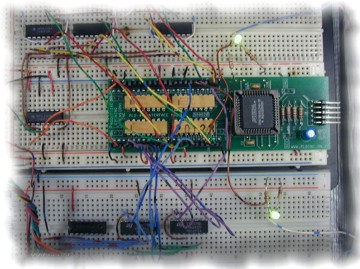
We
programmed our code using MAX+plus II 9.23 onto our EPLD. Our VHDL
code is shown below, with comments explaining the various cases we covered. For another form of visual representation, please see
the animated
simulation.
VHDL File
--
Code Start --
library ieee;
use
ieee.std_logic_1164.all;
use
ieee.std_logic_unsigned.all;
entity
backup is
port
(clk, clkcount, car_on, car_moving : in
std_logic;
distance, msd : in
std_logic_vector (3 downto 0);
intalarm1, intalarm2,
intalarm3 : out std_logic;
extalarm1, extalarm2,
extalarm3 : out std_logic;
lights1, lights2,
lights3 : out std_logic);
end
backup;
architecture backup_arch of backup is
signal
last_distance : std_logic_vector (3 downto 0);
--keep track of distance value for
comparison
signal
count : std_logic_vector (4 downto 0);
signal
countplus : std_logic;
signal
speed : std_logic_vector (1 downto 0);
begin
process (clk)
begin
if clk'Event and
clk = '1' then
count <= count + 1;
if count = "11111" then
countplus <= '1';
-- this will indicate a long period of
time has passed
end if;
if msd = "0000"
and distance <= "0011"
then
-- if the distance is less than 40 inches
if last_distance = distance then
-- if distance has not changed
if count = "11111"
then
-- if count is full
--if countplus <= '1' then
-- enough cycles have passed, turn off alarms
--intalarm3 <= '0';-- this portion of the code did not fit on
the EPLD
--intalarm2 <= '0';
--intalarm1 <= '0';
--extalarm3 <= '0';
--extalarm2 <= '0';
--extalarm1 <= '0';
--lights3 <= '0';
--lights2 <= '0';
--lights1 <= '0';
--else
countplus <= '1';
--end if;
end if;
elsif last_distance > distance then
-- action phase... distance has decreased
if countplus = '1' then
-- if long time has passed
speed <= "01";
-- speed is now marked slow
countplus <= '0';
else
if count <= "00010"
then
-- if short time has passed
speed <= "0011";
-- speed is now marked fast
elsif count <= "01010"
and count >"00010"
then --
if count in mid range
speed <= "10";
-- speed is marked medium
else
speed <= "01";
-- otherwise speed is slow
end if;
end if;
count <= "00000";
-- reset counter
if car_on = '1'
then
if car_moving = '1'
then
--
CASE 1: Car on, moving (backing into object)
if distance = "0000"
or speed = "11" then
-- distance <10 or fast speed
intalarm3 <= '1';
intalarm2 <= '0';
intalarm1 <= '0';
elsif
distance = "0001" or
speed = "10" then
-- distance >= 10 and <20,
--
or medium speed
intalarm3 <= '0';
intalarm2 <= '1';
intalarm1 <= '0';
else
-- distance >=20 and slow speed
intalarm3 <= '0';
intalarm2 <= '0';
intalarm1 <= '1';
end if;
else
-- CASE 2: Car on, not moving (hit from
behind)
if distance = "0000" or
speed ="11" then
-- distance <10 or fast speed
intalarm3 <= '1';
intalarm2 <= '0';
intalarm1 <= '0';
end if;
end if;
else
-- CASE 3: Car off (somebody is too
close)
if distance = "0000" or
speed = "11" then
-- distance <10 or fast speed
extalarm3 <= '1';
extalarm2 <= '0';
extalarm1 <= '0';
lights3 <= '1';
lights2 <= '0';
lights1 <= '0';
elsif
distance = "0001" or speed =
"10" then
-- distance >=10 and <20, or medium
speed
extalarm3 <= '0';
extalarm2 <= '1';
extalarm1 <= '0';
lights3 <= '0';
lights2 <= '1';
lights1 <= '0';
else
-- distance >=20 and slow speed
extalarm3 <= '0';
extalarm2 <= '0';
extalarm1 <= '1';
lights3 <= '0';
lights2 <= '0';
lights1 <= '1';
end if;
end if;
else
-- Distance has increased, reset alarms
and counter
intalarm3 <= '0';
intalarm2 <= '0';
intalarm1 <= '0';
extalarm3 <= '0';
extalarm2 <= '0';
extalarm1 <= '0';
lights3 <= '0';
lights2 <= '0';
lights1 <= '0';
count <= "00000";
end if;
else
-- Distance >39 inches, reset alarms
intalarm3 <= '0';
intalarm2 <= '0';
intalarm1 <= '0';
extalarm3 <= '0';
extalarm2 <= '0';
extalarm1 <= '0';
lights3 <= '0';
lights2 <= '0';
lights1 <= '0';
end if;
end if;
end process;
Process (clkcount)
begin
if clkcount'Event and
clkcount = '1' then
last_distance <= distance;
end if;
end process;
end
backup_arch;
--
Code End --
|